2 Getting Started - Reference Documentation
Authors: groovyquan
Version: 0.5.1
Table of Contents
2 Getting Started
2.1 Hello World
DOCTYPE
The html pages generated by gsp must generate the doc type as follows.<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
Importing resources
Add the tag <z:resources/> to the head of the layout/main.gsp or any page that you want to use ZK UI Grails plug-ins.<html xmlns="http://www.w3.org/1999/xhtml"> <head> <z:resources/>
Hello World!
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Hello World Demo</title> <z:resources/> </head><body> <z:window title="My First ZK UI Application" border="normal"> Hello World! </z:window> </body> </html>
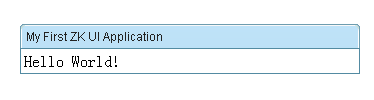
2.2 Say Hello in Ajax way
Let us put some interactivity into it.<z:button label="Say Hello" onClick="Messagebox.show('Hello World! Time now is:'+new Date())"/>
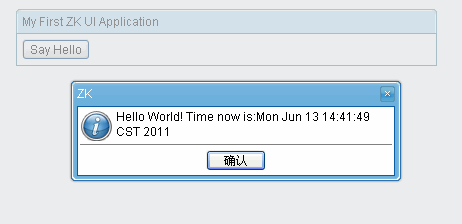
Notice that it is not JavaScript,It is Groovy and runs at the server
2.3 Identify a component
A component is a POJO, so you can reference it any way you like. However, ZK provides a convenient way to identify and to retrieve a component: identifier. For example, we named the textbox as input by assigning id to it, as follows.<z:window title="Property Retrieval" border="normal"> Enter a property name: <z:textbox id="input"/> <z:button label="Retrieve" onClick="Messagebox.show(System.getProperty(input.getValue()))"/> </z:window>
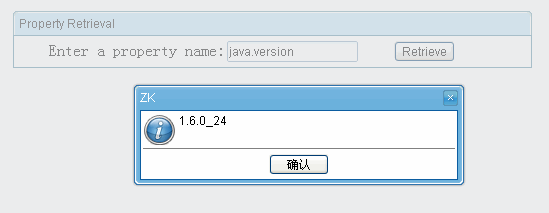
2.4 A component is a POJO
A component is a POJO. You could instantiate and manipulate directly. For example, we could generate the result by instantiating a component to represent it, and then append it to another component (an instance of vlayout).<z:window title="Property Retrieval" border="normal"> Enter a property name: <z:textbox id="input"/> <z:button label="Retrieve" onClick="result.appendChild(new Label(System.getProperty(input.getValue())))"/> <z:vlayout id="result"/> </z:window>
<z:window title="Property Retrieval" border="normal"> Enter a property name: <z:textbox id="input"/> <z:button label="Retrieve" onClick="result.setValue(System.getProperty(input.getValue()))"/> <z:separator/> <z:label id="result"/> </z:window>
2.5 Separating code from user interface
Embedding Groovy code in a gsp page is straightforward and easy to read. However, in a production environment, it is usually better to separate the code from the user interfaces. In additions, the compiled Groovy code runs much faster than the embedded code which is interpreted at the run time.To separate code from UI, we could implement a Composeruse create-composer commandgrails create-composer zkuidemo.PropertyRetriever
package zkuidemoimport org.zkoss.zk.ui.Component import org.zkoss.zk.ui.event.Event import org.zkoss.zul.Labelclass PropertyRetrieverComposer { def afterCompose = {Component target -> target.addEventListener("onClick", new org.zkoss.zk.ui.event.EventListener() { //add a event listener in Groovy public void onEvent(Event event) { String prop = System.getProperty(target.getFellow("input").getValue()) target.getFellow("result").appendChild(new Label(prop)) } }); } }
package zkuidemoimport org.zkoss.zk.ui.Component import org.zkoss.zk.ui.event.Event import org.zkoss.zul.Labelclass PropertyRetrieverComposer { def afterCompose = {Component target -> target.addEventListener("onClick") {Event event -> String prop = System.getProperty(target.getFellow("input").value) target.getFellow("result").appendChild(new Label(prop)) } } }
<z:window title="Property Retrieval" border="normal"> Enter a property name: <z:textbox id="input"/> <z:button label="Retrieve" apply="zkuidemo.PropertyRetrieverComposer"/> <z:separator/> <z:vlayout id="result"/> </z:window>